Along with user interface testing and feature testing, confirming your app’s API works as it should is integral to the successful launch of your product. A buggy API would mean your app is unable to communicate correctly with other systems. Testing before and after release can eliminate these issues that could plague your users.
Manual API testing can be time-consuming, but fortunately there are tools to automate and ultimately speed up the testing process. This article discusses the importance of API testing and how to use Postman to create easy-to-use, repeatable API tests.
What is API testing?
An API, or application programming interface, is what your app, program, or service uses to communicate with other apps, programs, or services. This is a way for your app to pull information from a database or website, or give information to an app requestion information.
These days, web applications are built in an API + UI fashion, so you don’t need to build a monolithic “web app” as before. Now, you would separately build an API and then also build a UI on top of the API.
APIs work behind the scenes and transport only raw data, since there’s no human interface that would require the data to be presented in a visually appealing way. This allows for the fast transmission of information while still providing everything your app, program, or service needs to operate.
API testing is a means of verifying that two software systems, which could also be your API and own UI are able to talk to each other without issue. It’s a way of checking the performance, reliability, and functionality of your app’s API.
As mentioned before, API testing ignores the graphical interface that a user would touch and focuses entirely on the application layer (OSI model) of your software. API tests use calls sent from an automation tool to your app’s API. The automation tool receives back the API’s output and records the information it receives, which can be checked against an expected result.
What is Postman?
Postman is an automation and development tool that you can use to build an API for your app and automate the tests you need to make sure the API is working correctly.
With Postman, you can take tests that you would typically have to perform manually and run them through an automated system. The platform enables you to perform unit tests, integration tests, functional tests, mock tests, end-to-end tests, regression tests, and more.
Software and QA engineers use Postman to create test suites that they can reuse at different phases of development to ensure the absence of bugs in the software.
Likewise, Postman frees teams up from the burden of manual testing, allowing them to focus on critical project areas like feature implementation. Automated testing also removes human error that could confound test results .
Postman has an app for Windows, Mac, Linux, and can be used through a Chrome browser. Using Postman is as simple as downloading and installing the app.
What are Postman collections?
Postman collections is a tool within the Postman platform that organises your API requests. As Postman runs requests to check your software’s API functionality, those requests and their results are saved under your history tab. Over time and with multiple projects in development, the history tab can become difficult to sift through.
Collections allows you to group your requests by project, type, or in any way of your choosing. As you create new requests, Postman allows you to conveniently save a request directly to the collection in which you’d like to store it You can go into your collections at any time to see request results or run those requests again.
Many frameworks and software services such as Mailosaur have collections of their own that you can fork (copy) through your Postman account.
Now that you have a basic understanding of Postman and its testing capabilities, let’s go ahead and create a simple API test.
Creating a simple API test with Postman
Handling requests is only a small part of what Postman can do. While a request is a way to send or receive data from your app’s API, you’ll need to write tests with pass/fail criteria that will tell Postman if your API is doing what it was designed to do.
Tests in Postman are written in JavaScript using the “Tests” tab in the program. Once you’ve written your code, you can use Postman to either GET data from your API, POST data to your API, or use other methods such as PUT, COPY, and DELETE. Postman will run the test based on the criteria defined in your code.
The following example uses Postman’s echo API (https://postman-echo.com) as our test source. This echo service simply sends back what it receives, so results are easy to estimate. We can add parameters test1
and result1
which the echo API will return to us as results.
Typing https://postman-echo.com/get?test1=result1 into Postman as the API we’re going to GET data from allows us to create some tests to verify that our API sending the correct information. In this case, since we’re sending test1
and result1
to the server, we can assert that the echo server will send the same values back.
Clicking on the “Tests” tab, we can add our JavaScript test scripts into Postman. A simple test using the GET method should have formatting similar to the script below:
pm.test("Name of the first test", function () {
// make an assertion
// if your assertion throws an error, this test will fail
pm.response.to.have.status(200);
});
pm.test("Name of the second test", function () {
// make as many assertions as you'd like as part of this test
// if any assertion throws an error, this test will fail
pm.response.to.have.status(200);
pm.response.to.be.ok;
pm.response.to.be.json;
});
A pm.test
, or Postman test, is the syntax used to create a test in Postman. Each pm.test
must have a name that outlines what the test is checking and a function with an assertion listing what we expect to see as the output from an API.
In our example, we assert that the output from the Postman echo API will be test1
and result1
. If these are indeed the outputs Postman receives from the API, Postman will declare a status code ‘200’, which simply means that Postman has received the expected value.
To demonstrate this in Postman, we can write a test called “Status code is 200” that will pass if the status code comes back as ‘200’. Should we assert that we expect a different status code (such as ‘500’), we can create a test for that as well. We can also write a test to have Postman verify that API output matches a particular string.
Postman allows us to perform multiple tests at the same time within the same call. Therefore, we can list the following three tests together and run them concurrently:
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
pm.test("Status code is 500", function () {
pm.response.to.have.status(500);
});
pm.test("Body matches string", function () {
pm.expect(pm.response.text()).to.include("result1");
});
Two of our tests pass without issue. Since Postman returns a status code of ‘200’ and not ‘500' as we asserted in test two, this test fails. The text below reveals what Postman’s test results show us:
PASS Status code is 200
FAIL Status code is 200 | AssertionError: expected response to have status code 500 but got 200
PASS Body matches string
This is how the result looks in the Postman user interface:
Advanced Testing Tips
Testing an endpoint that results in an email or SMS notification can cause issues with API testing. Unfortunately, testing such functionality with Postman is not possible because you cannot validate whether hitting an endpoint in a Postman API test results in an email or an SMS being sent.
For email and SMS tests, you can use Postman API tests alongside Mailosaur. The latter offers an API (and a collection) that can be combined with Postman to check how email or SMS notifications are working with your app.
Once you’ve worked your way through a few simple tests, you’ll want to take your Postman test writing to the next level. Postman has several guides on their site that teach you to do just that.
Use Mailosaur’s API and Postman collection to perform email and SMS testing
To perform email and SMS testing with Postman at the API level, look no further than Mailosaur’s API to send and receive messages. Having a Mailosaur account enables your whole team to ass tests for email and SMS. Mailosaur even has a forkable Postman collection that you can add directly to your workspace here
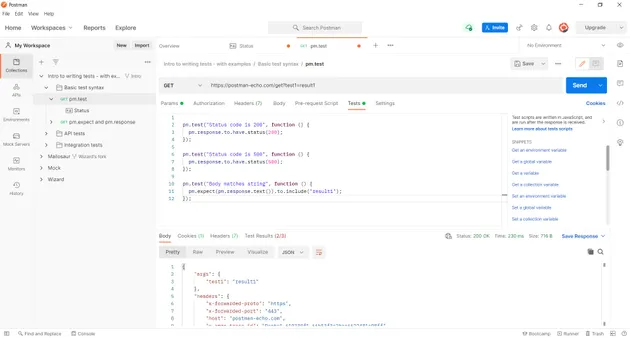
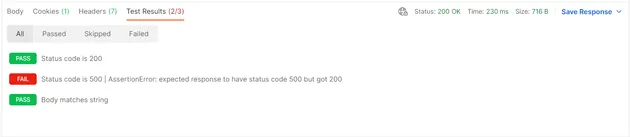